Quickstart
This Quick start guide will take you through the process of retrieving necessary tokens for authentication, linking a customer's bank accounts to Link Money's services, and initiating payments. By the end of it, you will have covered Link Money's primary user experience.
At a Glance
Once Link Money has granted you merchant portal access at support@link.money you will have all the information necessary to integrate the Pay by Bank product. The two primary integration components are:
- Session API
- Frontend SDK
To begin, you initiate a session by calling the Session API, providing the necessary parameters (STEP 1). This API call returns a session key along with a session URL that needs to be sent to the client. Using the Link frontend SDK, we direct the client to this URL where they link their bank account and complete the payment transaction (STEP 2). After the process concludes, a callback is triggered, redirecting the client back to a designated URL on your side, signaling completion of the transaction.
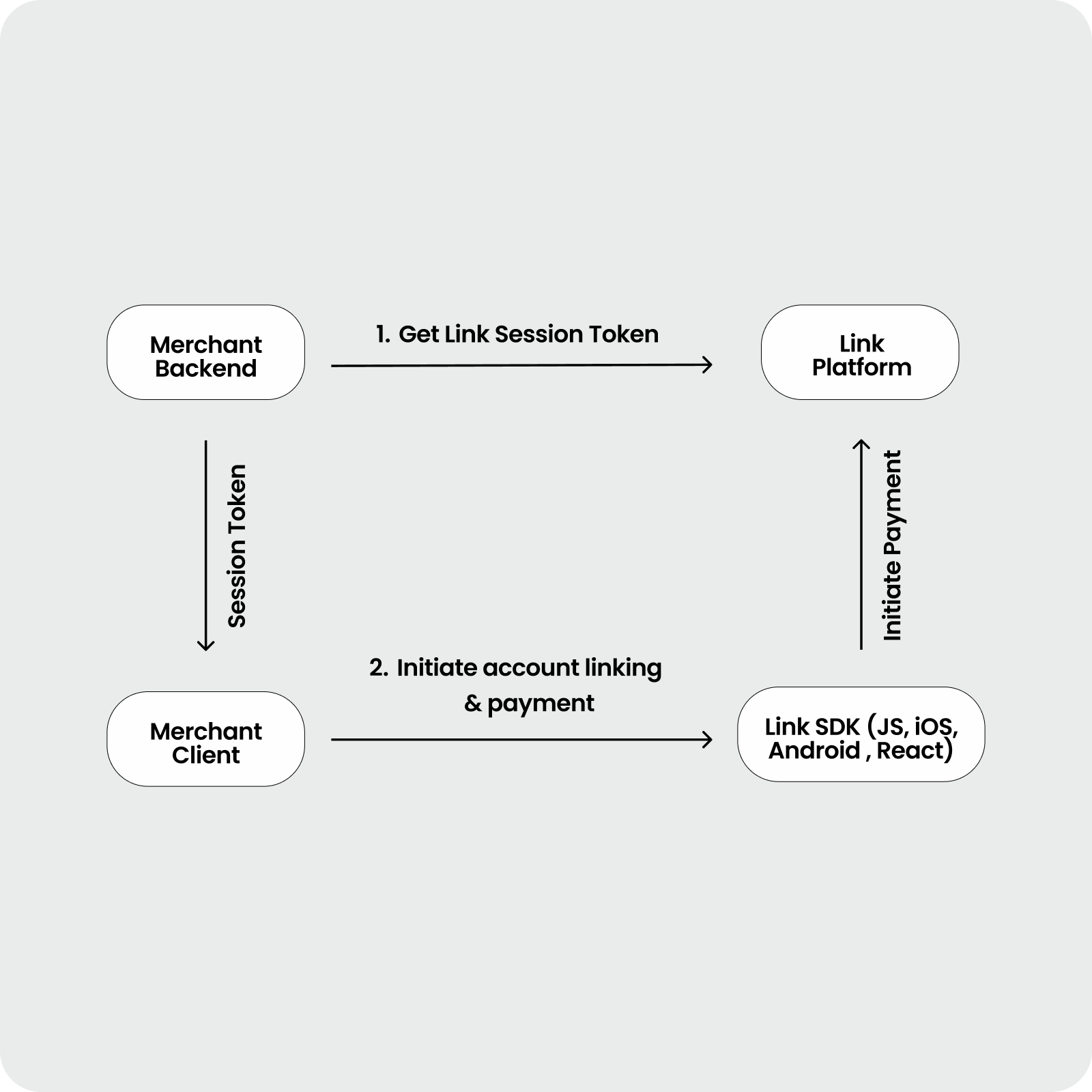
1. Get Session Key
All information required to link accounts and initiate payments will be communicated through this API. You will need to provide a the ClientID & Secret found in the merchant portal as a header. To gain access to the portal work with your Merchant Admin or request access directly from the Link Money team.
This endpoint returns a session key and a URL to be used for SDK requests. The only mandatory fields in the session request are email, firstName, and lastName. Additional data provided through the sessions API enables the payment functionality, maximizes our ability to decision payments and enhance the user experience; see the Creating Sessions section.
1curl --location --request POST '{API_BASE_URL}/v2/sessions'
2--header 'Content-Type: application/json'
3--header 'Accept: application/json'
4--header 'Authorization: Basic <base64({CLIENT_ID}:{CLIENT_SECRET})>'
5--data-raw '{
6 "firstName" : "{CUSTOMER_FIRST_NAME}",
7 "lastName" : "{CUSTOMER_LAST_NAME}",
8 "email" : "{CUSTOMER_EMAIL}",
9 "orderDetails": {
10 "totalAmount": { "value": float, "currency": "USD" },
11 },
12 "customerProfile":{
13 "id": string,
14 "guestCheckout": boolean,
15 },
16 "paymentDetails": {
17 "amount": {
18 "value": float,
19 "currency": "USD"
20 },
21 "requestKey": string,
22 "softDescriptor": string,
23 "clientReferenceId": string
24 },
25 "product": enum,
26 "redirectUrl": string,
27 "experienceId": enum
28 }'
29
Returns
sessionKey String
A session key which establishes a trust between customer and Link
sessionUrl String
URL required for customer to be directed for linking and payment
*The sessionKey and sessionUrl are required and used in step 2: Frontend SDK
Response Body
{
"sessionKey" : "a5292de413e-2626d8244239-879a9-ffbdfa2",
"sessionUrl": "https://client.link.money?redirect={REDIRECT_URL}&version=web-v1.0.0&sessionKey={SESSION_KEY}"
}
2. Frontend SDK
The SDK is used to link customer bank accounts and initiate payments. The session key and the sessionUrl provided in the previous step are required to make this request. The production redirect URL must be pre-approved by Link Money to avoid malicious exploitations.
This step can be found in the SDKs page along with other ways of integrating the products functionality.
The SDK will return all customer and transaction information necessary to interact with the Link Money API suite in the redirect URL.
1<html>
2 <body>
3 <div id="btnContainer"></div>
4 <script>
5 import Link from 'https://static.link.money/linkmoney-web/v1/latest/linkmoney-web.min.js';
6 const config = {
7 sessionUrl: '{SESSION_URL_FROM_SESSION_CALL}';
8 environment: 'production'|'sandbox',
9 sessionVersion: 2,
10 };
11 const link = Link.LinkInstance(config);
12 document.getElementById('btnContainer').appendChild(link.createButton());
13 </script>
14 </body>
15</html>
Returns
status number
Indicates the Bank Linking status
customerId String
Newly generated customer ID. You must retain this value to use for payments and additional APIs
paymentId String
Link Money-generated payment-related ID
paymentStatusCode number
Indicates the status of the transaction
status - Possible Values
- 200: The customer successfully linked a bank account and payment request successfully created
- 204: Customer exited voluntarily
- 500: Error occurred
paymentStatusCode - Possible Values
- 0: AUTHORIZED - Transaction was authorized for payment
- 1: TERMINAL_FAILED - Transaction did not complete successfully or was declined
- 2: PENDING - Transaction is pending further review
Response Body
{REDIRECT_URL}/?status=200&customerId={CUSTOMER_ID}&paymentId={PAYMENT_ID}&paymentStatusCode=0
Putting it all together
- Please review the Integration section to identify various ways your integration can be tested. You will also find base URLs needed for invoking API calls.
- Link Money recommends that your client ID and client secret provided by Link Money should not be exposed outside of your backend. All authorization tokens should be confined to your backend as well.
- It is expected that you will implement an API endpoint for your frontend to obtain the session attributes to pass on to Link Money’s SDK
- The endpoint must be an authenticated endpoint, i.e. your frontend must pass whatever authentication you use to verify the request
- It is also important that your session key endpoint only allows obtaining a session key for a specific customer
- You can initiate a payment in one of three ways depending on your desired user experience
- If the payment is initiated by the customer through the Link Money UI, you will not need to integrate the payments endpoint and will pass payment information directly at session creation
- If the payment is initiated by the customer through your UI, you will need to implement a payment initiation endpoint with authorization on your backend which in turn calls Link Money’s payment API
- If payment is initiated by a backend process, such as a scheduled or recurring payment, your backend can directly invoke Link Money's payment API
- To enable full payment functionality you will need to subscribe to payment webhooks
- Refunds can be handled through the merchant portal but should be handled with the refund API